Dynamics BC API Client
The API client for communicating with Dynamics BC was designed such that structured objects are used to transfer data between Magento and Dynamics BC. The client subsystem consists of multiple components that interact with each other and introduce abstraction layers to solve recurring problems.
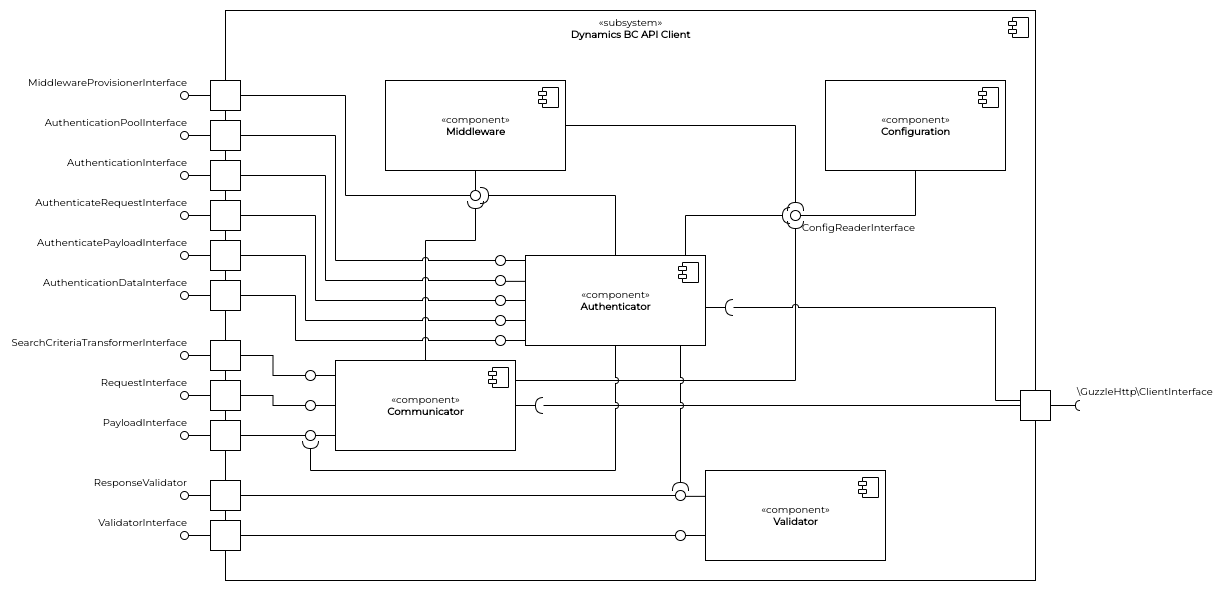
As can be seen in the component diagramm above, the subsystem consists of the following components which have clearly defined responsibilities and may be accessed by the interfaces they expose only.
Component | Responsibility |
---|---|
Authenticates the requests created by Communicator using an extensible set of authentication mechanisms. |
|
Performs the actual communication with Dynamics BC. Use the requests exposed by this component to send and receive data. |
|
Allows the other components to read configuration options. |
|
Applies an extensible set of default query parameters to the requests sent b the client. |
|
Provisions the client used by requests with an extensible set of middleware (e.g. request logging, authentication, application of default parameters). |
|
Provides an extensible set of validators that can be used to validate the responses returned by Dynamics BC (e.g. HTTP status, content type). |
Authenticator
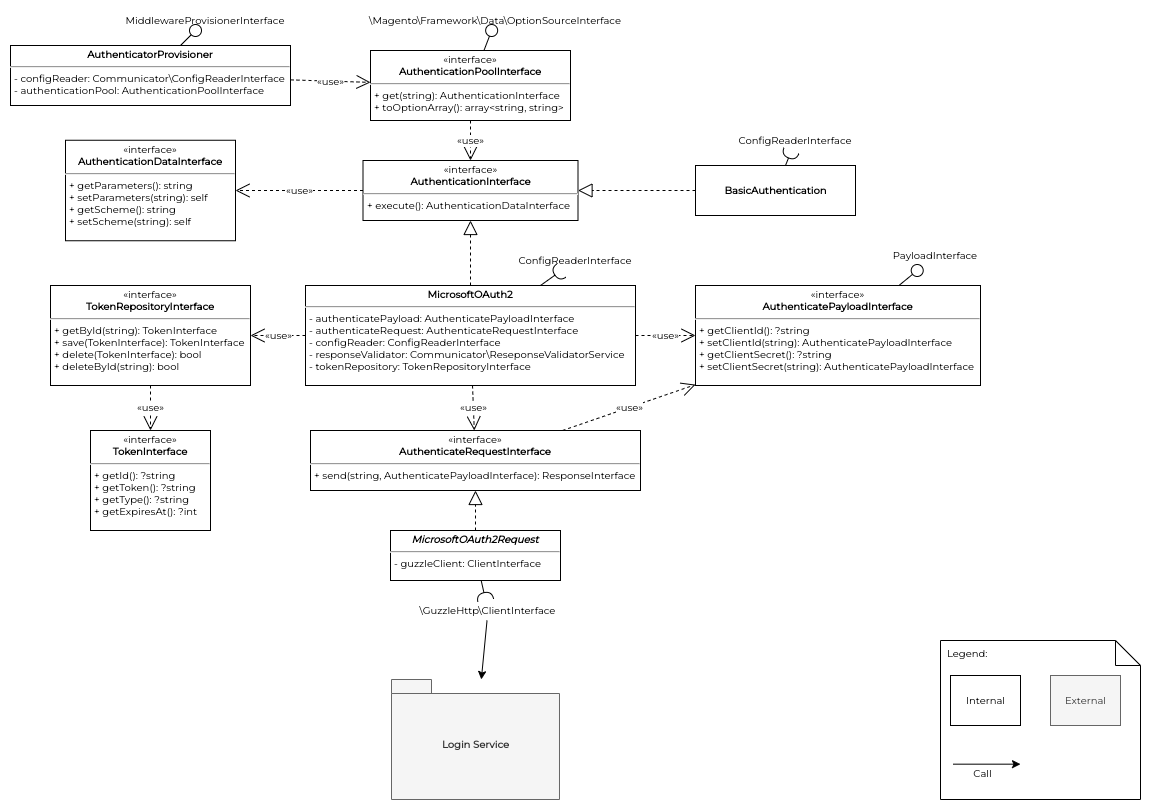
The Authenticator component is responsible for providing authentication mechanism and allowing to extend the list of such mechanisms by external modules.
By default, it comes with the following mechanisms:
Mechanism | Class name | Explanation |
---|---|---|
HTTP Basic Authentication |
|
Uses the configured username and password to perform basic authentication of requests. |
Microsoft OAuth2 service |
|
Uses the configured username and password, as well as some specific configurations to acquire a temporary bearer token that authenticates requests. |
The list of mechanisms can be extended by implementing the exposed interfaces AuthenticationInterface
& AuthenticationDataInterface
and adding it to the exposed AuhenticationPoolInterface
via dependency injection.
Communicator
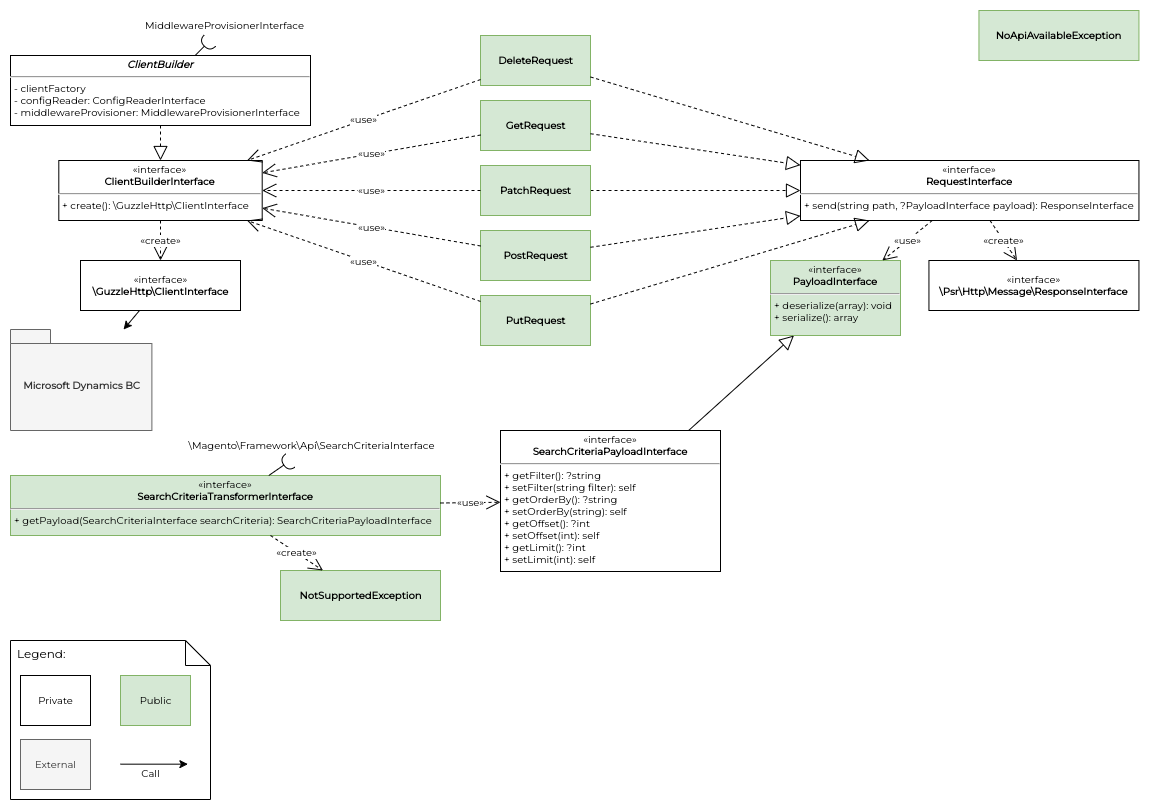
This component provides all the classes needed to communicate with Dynamics BC. In order to send or retrieve data to / from Dynamics BC, a module must make use of the public implementations of RequestInterface
.
In order to send data to Dynamics BC, a module must provide an implementation of PayloadInterface
to the request.
The purpose of PayloadInterface
is to be able to provide specialized Data transfer objects (aka DTOs), meaning that a module must not directly implement PayloadInterface
but rather provide an extending interface (and an implementation of the latter) that defines the accessors and mutators of a specific DTO (as demonstrated by SearchCriteriaPayloadInterface
).
Dynamic BC API pages support filtering and sorting of results through special query parameters. Since Magento developers are familiar with using \Magento\Framework\Api\SearchCriteriaBuilder
in order to filter and sort results, the default implementation of the public interface SearchCriteriaTransformerInterface
provides a facility to transform Magento’s search criteria into filter and sorting conditions understood by Dynamics BC.
The result of SearchCriteriaTransformerInterface::getPayload()
implements PayloadInterface
and can thus be passed to any request (typically it will be passed to a GetRequest
).
In case a filter condition cannot be translated to Dynamics BC or is not supported by the latter, a NotSupportedException
is thrown.
The public exception NoApiAvailableException
must be used to indicate that a specific remote operation is not supported by Dynamics BC (e.g. deletion of a customer).
Configuration
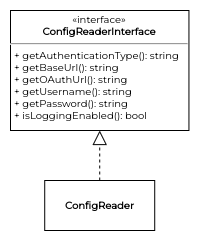
This component provides a facility to read settings from the system configuration that are related to the whole subsystem. It must not be used by external modules. In case an external module needs configurations provided by the subsystem (e.g. username, password), then it has to use its own configuration reading mechanisms.
Default query parameter applicator
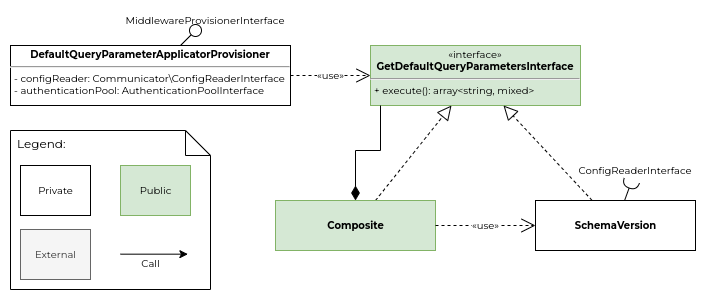
This component was introduced in version 1.2.0
of the package. Its purpose is to apply an arbitrary amount of query parameters to any request sent by the client.
In order to add custom query parameters to the set of default query parameters, an implementation of the interface GetDefaultQueryParametersInterface
must be created and injected into the public class Composite
.
Middleware
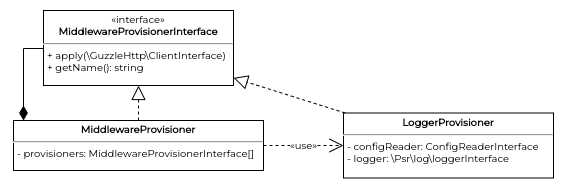
This component provisions middleware on the client created by the Communicator component. The class MiddlewareProvisioner
acts as a composite that applies all implementations of MiddlewareProvisonerInterface
that are injected into its constructor on the client stack.
In order to extend the list of middleware applied to the stack, an external modul has to implement MiddlewareProvisonerInterface
and add this implementation to MiddlewareProvisioner
using dependency injection.
Validator
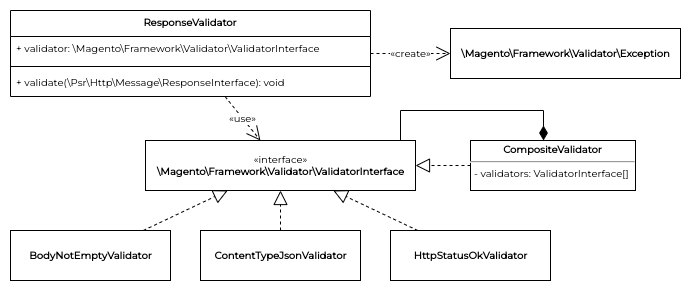
This component provides validators that can and should be used when inspecting the response of a request. All validators that are implemented here are exposed and can thus be used by external modules.
The following validators already exist:
Class name | Explanation |
---|---|
|
Validates that the body of the response has a size greater than zero. |
|
Executes all validators that are injected into its constructor and returns whether all of them were successful. |
|
Validates that the response had a |
|
Validates that the HTTP status code of the response is |
A virtual type derived from CompositeValidator
, containing all the validators mentioned above, is injected into the service class ResponseValidator
, such that the latter can be used to perform basic response validation.
In order to implement custom validators, an external module may implement the interface \Magento\Framework\Validator\ValidatorInterface
and create a virtual type derived from ResponseValidator
into which the custom validator is injected.