Function descriptions
PipelineInitializer
The integrated ScheduleEntitiesForExport
and RescheduleErroredEntitiesForExport
of the Order Entity Export implement the
InitializationDataFetcherInterface
of the Process Pipeline
component to create pipelines with the heartbeat dynamically.
<?php
public function execute(): array
{
$result = [];
foreach ($this->exportEntityDefinitionList->get() as $entityDefinition) {
if (!$entityDefinition->isActive()) {
continue;
}
$entityIds = $this->retrieveSchedulableEntities->execute(
$entityDefinition->getEntityTableName(),
$entityDefinition->getEntityIdentifierFieldName(),
$entityDefinition->getCode()
);
foreach ($entityIds as $entityId) {
$initializationData = $this->initializationDataFactory->create();
$initializationData->setArguments(
[
self::ARGUMENT_ENTITY_CODE => $entityDefinition->getCode(),
self::ARGUMENT_IDENTIFIER => (int)$entityId,
self::ARGUMENT_IS_RETRY => false,
]
);
$initializationData->setPipelineConfiguration(
$this->getPipelineConfiguration(
$entityDefinition,
(int)$entityId
)
);
$result[] = $initializationData;
$this->markEntityAsScheduled->execute(
$entityDefinition->getCode(),
(int)$entityId
);
}
}
return $result;
}
All orders not already scheduled for export are determined and filtered based on the configuration
- Identification of the orders to get exported is filtered by the modifiers and executed here:
-
-
\TechDivision\PacemakerEntityExport\Model\ResourceModel\RetrieveSchedulableEntities::execute
-
TechDivision\PacemakerEntityExport\Model\ResourceModel\RetrieveReschedulableErroredEntities::execute
-
<?php
/**
* @param string $entityTableName
* @param string $entityIdentifierFieldName
* @param string $entityCode
* @return array
*/
public function execute(string $entityTableName, string $entityIdentifierFieldName, string $entityCode): array
{
$connection = $this->resourceConnection->getConnection();
return $connection->fetchCol(
$this->modifier->execute(
$entityTableName,
$entityIdentifierFieldName,
$entityCode,
$connection->select()
)
);
}
-
Information about the export status of the order is held in the `pacemaker_entity_export' database table
-
Once an order entity has been identified, a pipeline is dynamically created using the appropriate pipeline definition
- The required interface for each entity pipeline definition is:
-
-
TechDivision\PacemakerEntityExportInitializer\Model\ExportEntityDefinitionInterface
-
- The following information is specified in the Order Entity Pipeline Definition:
-
-
Code: The code for this entity; here: order_export
-
Table name: The name for the main table; here: sales_order
-
Identifier field name: The name for the ID field; here: entity_id
-
Pipeline config: The pipeline configuration for this entity pipeline. Can be defined with xml or dynamically
-
Is active: Configuration when the entity definition is active
-
The update of the order or the order status takes place in the steps of the pipeline.
The external ID is set by the ResponseHandler
as soon
as the target system
(OMS/ERP)
has received/processed the order.
ExportFormatProvider
-
The
ExportFormatProvider
is a pipeline step executor used dynamically for the transform step -
The configuration is used to define the export format
-
As default, the Custom Template is used, which is editable in the backend with a Twig editor
-
Several export formats can be registered beside the Custom Template
TransportAdapterProvider
-
The
TransportAdapterProvider
is a pipeline step executor that is used dynamically for the transport step -
It gets used to define the export functionality and workflow
-
By default, the file system gets used
-
Besides the file system, other transport paths can get registered, e.g., through API or DB
ResponseHandlerProvider
-
The
ResponseHandlerProvider
is a pipeline step executor used dynamically for the handle response step -
The configuration manages responses regarding receipt and processing of a target system (OMS/ERP)
-
By default, a JSON file gets expected on the target directory of the file system, which has a reference to the external id
-
Besides the default JSON format, other formats like XML can get registered
-
Managing the responses is optional
NotificationHandlerProvider
-
The
NotificationHandlerProvider
is a pipeline step executor used dynamically for the handle notification step -
The configuration gets used to notify about responses and information
-
In addition to the default email notification, other notifications such as loggers can get registered
-
The notifications are optional and disabled by default
Reservation Handling
With the Reservation Handling feature in Pacemaker Order Export, you can easily configure when inventory levels are adjusted with respect to product stock reservations.
If Pacemaker Order Export is configured to cancel the reservations through Pacemaker, then a separate step is created in the pipeline to perform this stock/reservation cancellation during execution.
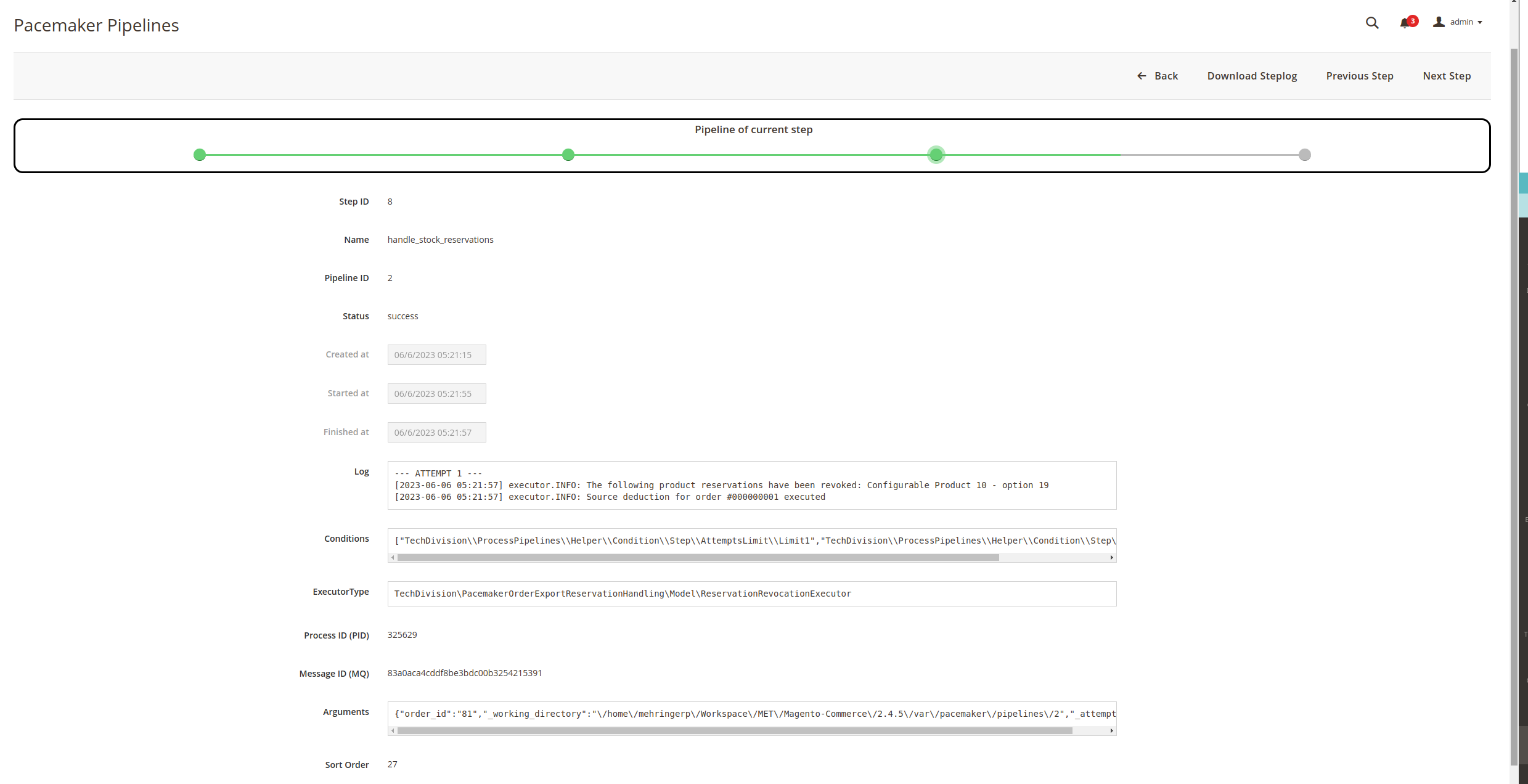
Technically, the inventory is corrected and the reservation is cleared by an offsetting entry.

- The following configuration settings are available::
-
=== Use Magento Standard
Magento takes care of the inventory/reservation cancellation when creating shipments, credit memos or cancellations.
Pacemaker does not execute any logic and does not generate a step in the order export pipeline.
After Transport Step
Here, Pacemaker steps in to handle the reservation.
After the transport step (export), a step is created in the order-export pipeline.
-
This step ensures that the reservation of the ordered products is resolved after the export
The system only checks whether the transport step was executed, not its status.

Depending on successful Transport Step
This option is similar to After Transport Step, but also checks for successful transport step status.
Depending on the export response
This option will generate the Reservation Processing Stage after the Reply Stage, rather than after the Shipment Stage. This is also checked for successful processing.
A response handler must get configured. |

Order Status Payment Adjustment
With the Order Status Payment Adjustment feature in label-pacemaker Order Export, it is easy to configure when the order should get canceled based on the payment method/status and a user-defined time.
When Order Status Payment Adjustment is configured to cancel orders that meet the conditions, a separate pipeline is created with a step to cancel orders during execution.
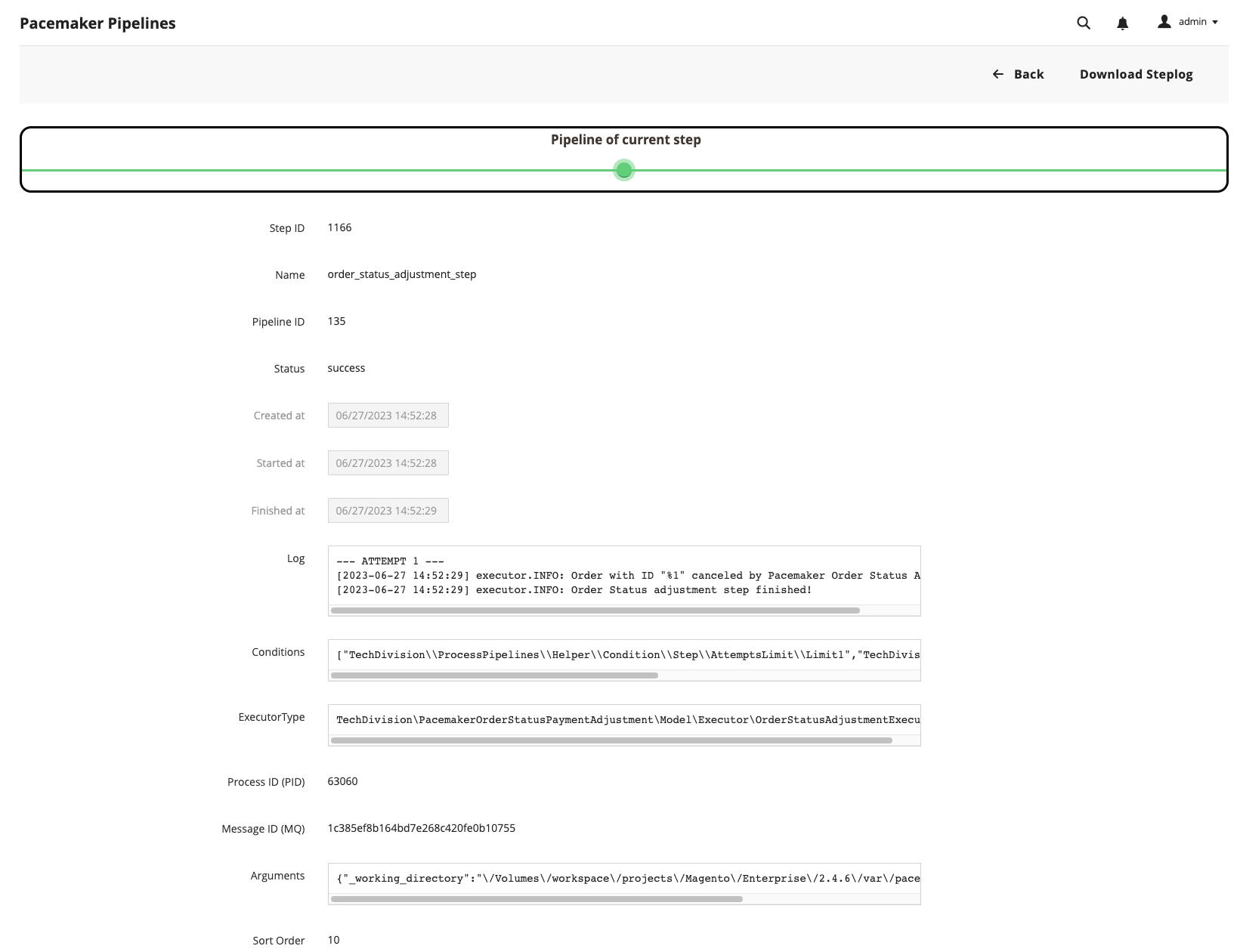
The status of the order gets set to canceled
.

The following four settings are available to configure order cancellation.
-
Only if all four settings apply to an order, it will get canceled
Payment Method
- The Payment Method setting restricts orders cancellation to those with the selected payment method:
-
-
Any payment method available in the store can be selected
-
Order Status to cancel
- This setting restricts orders cancellation to those with the selected order status:
-
-
It can get selected from the values
pending
andprocessing
-
Cancel order only if exported
This setting determines whether the cancellation of orders is limited to those exported by Pacemaker or whether it also includes orders that have not yet been exported.
If the option is set to Yes
, only orders listed in the pacemaker_order_export table with a value of 1
in the is_exported column will be considered.
Time Period
This setting limits the cancellation of orders to those whose creation date, when added within the specified time period, is less than the current date and time.
The period can get specified in minutes, hours, days, weeks, months, and years.
Scheduling the pipeline by cron expression
Pipeline creation can get scheduled using a cron expression.
By default, the pipeline is built at most once per minute.
If the heartbeat is executed twice per minute, by default the pipeline will only get built on the first execution and not on the second.
Any valid cron expression can get specified.
Pacemaker Pipeline Data Storage
The storage has been developed and integrated as a part of the export of the entity.
-
It is used to transfer data between steps
-
One step stores the information in a storage medium (default: database) and another step has access to it
-
The data storage is implemented with the adapter logic
-
Therefore, you do not necessarily have to rely on a database
-

Retry / Reset Mechanisms
Retry-Mechanism
The retry mechanism of the entity export is controlled via configuration and via the database table pacemaker-entity-export
. Errors are recorded during an export (try). If the number of errors is less than the configured value another export pipeline is created with the next heartbeat execution in order to export the entity, i.e. in this case the order.

If the number of attempts is reached, a new pipeline is no longer created. The option to reset the order remains.
Manual Reset via Admin Backend
If exports do not work without errors, e.g. if a third-party system cannot be reached and the configured number of possible failed attempts has been reached. It is possible to reset the export of the order in the backend in order to be able to execute the export function again
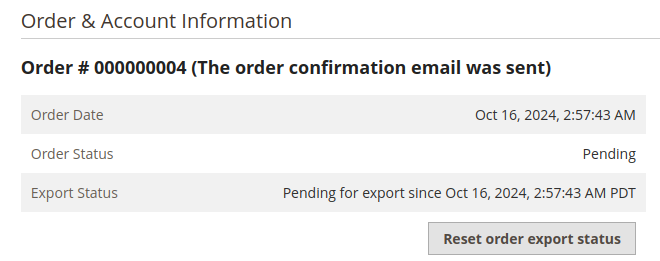
The button is only displayed if the data constellation of the order allows it in order to avoid data redundancies.
With the reset the status for the export is set to pending
and the error-count is reset to 0
.
Automatic Reset via Pipeline
It is also possible to manage the reset of order exports automatically (and manually) via a pipeline. This can be set via configuration.
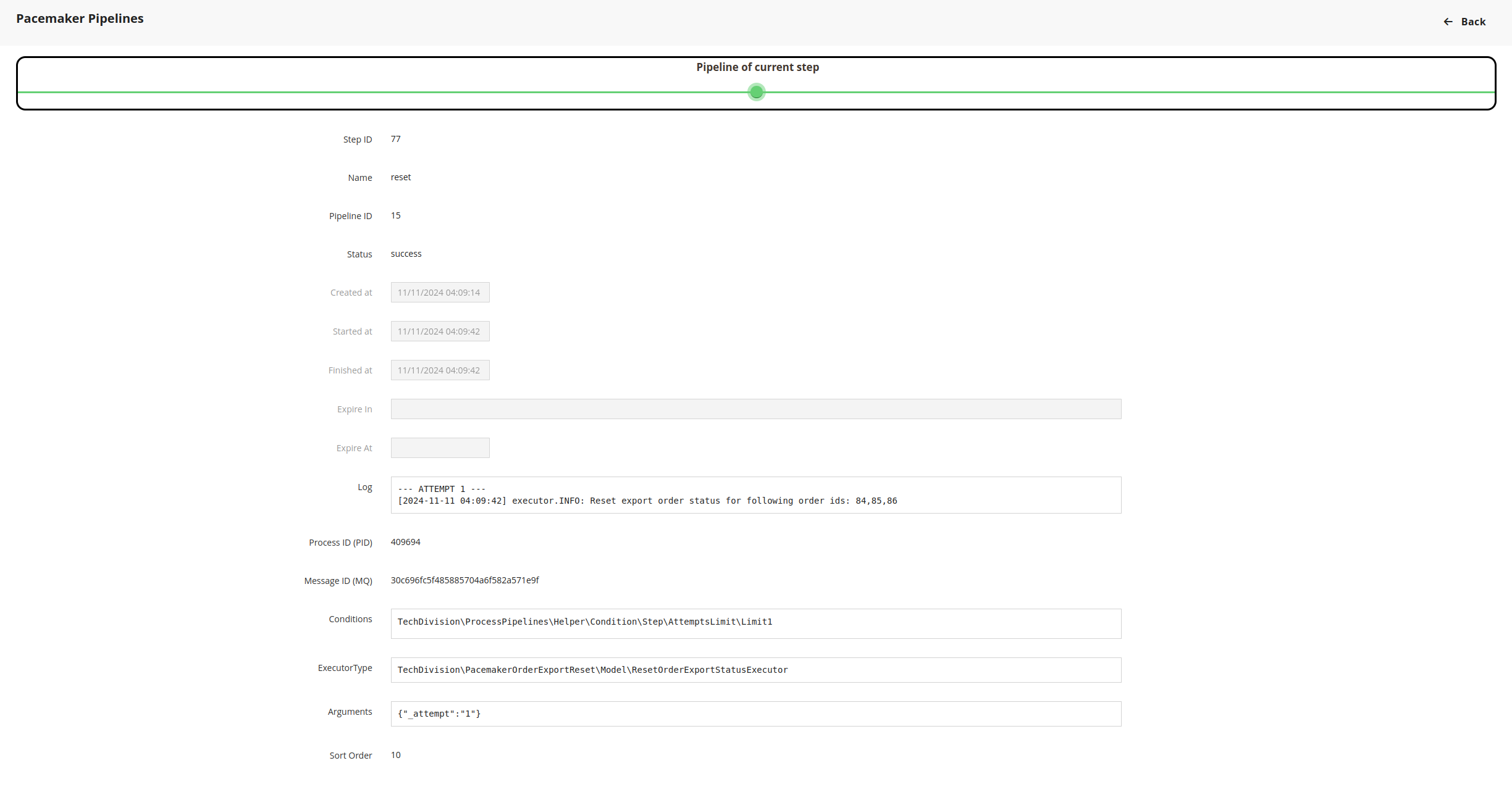
All exports with the status error
are determined via the pacemaker_reset_order_export
pipeline and checked to see whether the configured error count has been reached.
They are then reset in order to process the orders again in the standardised workflow.
Default Entity Export Modifiers
Entities are checked for export via the Entity Export and its Entity Export Initializer.
In addition to the functionality of scheduling exports, it also includes the retry mechanism. The check is carried out using so-called modifiers.
You can implement and register your own modifiers. Pacemaker offers the following standard modifiers, which you can use in your own implementation workflow:
Modifier | Description |
---|---|
BaseModifierComposite |
Serves as the basis of the Chain-Patter to register all necessary modifiers via DI. |
EntitySelectWithExportStatusJoin |
This is the initial select query to the database to load the central entity. |
WhereErrorCountIsLessThan |
A filter condition to determine the entities with fewer export failures than the configurable threshold. |
WhereLastErrorIsOlderThan |
A filter condition for the rescheduling process to initiate re-export attempts only after one hour. |
WhereStatusIsError |
A filter condition to get the export attempts with error status. |
WhereStatusIsPending |
A filter condition to get the export attempts with pending status. |
WhereOrderDatetimeFilterConfig |
A filter condition to load orders according to the configurable datetime setting. |
WhereOrderIsAssignedToWebsite |
A filter condition to load orders from the respective websites. |
WhereOrderPaymentAndStatusLikeConfig |
A filter condition to load orders according to the configurable payment status mapping. |
Data Migration for Pacemaker Entity Order Export Version 3.x to 4.x
A read-only config field is implemented with the update, which saves the date of the upgrade. This config ensures that only orders from the date with the new logic are exported.
You can view the config here: Pacemaker → Order Workflow → Order Export Settings → Integration/Update Date for the Entity Export
Furthermore, it is recommended that you configure the time period so that old orders are no longer exported.
When upgrading to the new major version, all order export pipelines in which the transport
step is running and enqueued will get canceled.
It also transfers to the new structure all orders with the status pending
that have not yet been exported.