Function descriptions
PipelineInitializer
The integrated PipelineInitializer
of the Order Export implements the
InitializationDataFetcherInterface
of the Process Pipeline
component to create pipelines with the heartbeat dynamically.
<?php
public function execute(): array
{
$results = [];
foreach ($this->storeManager->getWebsites(true) as $website) {
if (!$this->configuration->isEnabled($website)) {
continue;
}
$orders = $this->getNotScheduledForExportOrders->execute($website);
if (empty($orders)) {
continue;
}
$results[] = $this->initPipelinePerOrder($website, $orders);
$this->bulkUpdateExportStatus->execute(
$orders,
[
ExportStatusInterface::IS_SCHEDULED => 1,
ExportStatusInterface::SCHEDULED_AT => (new \DateTime('now'))
->setTimezone(new \DateTimeZone('UTC'))
->format('Y-m-d H:i:s')
]
);
}
if (!empty($results)) {
$results = array_merge(...$results);
}
return $results;
}
All orders not already scheduled for export are determined and filtered based on the configuration
\TechDivision\PacemakerOrderExport\Model\ResourceModel\GetNotScheduledForExportOrders::execute
<?php
/**
* Retrieve a list of order entity IDs for orders, which are not scheduled for export yet
*
* @param WebsiteInterface $website
* @return array
*/
public function execute(WebsiteInterface $website): array
{
$orderTable = $this->resourceConnection->getTableName('sales_order');
$paymentTable = $this->resourceConnection->getTableName('sales_order_payment');
$storeTable = $this->resourceConnection->getTableName('store');
$exportStatusTable = $this->resourceConnection->getTableName('pacemaker_order_export');
$connection = $this->resourceConnection->getConnection();
$select = $connection->select()
->from(['sales_order' => $orderTable], ['entity_id' => 'sales_order.entity_id'])
->joinLeft(['export' => $exportStatusTable], 'sales_order.entity_id = export.order_id', [])
->joinLeft(['store' => $storeTable], 'sales_order.store_id = store.store_id', [])
->joinLeft(['payment' => $paymentTable], 'payment.parent_id = sales_order.entity_id', []);
$this->applyFilterConditionsForPaymentAndStatus($website, $select);
$this->applyFilterForCreatedAtDatetime($website, $select);
$select->order('sales_order.created_at DESC');
return $connection->fetchCol($select);
}
The information regarding the export status of the order gets held in the pacemaker_order_export
database table.
With each scheduled export order a pipeline is dynamically created using the function \TechDivision\PacemakerOrderExport\Model\GetExportPipelineDefinition::execute
.
The update of the order or the order status takes place in the steps of the pipeline.
For this purpose, the export status gets set by the TransportAdapter
after the actual export.
The external ID is set by the ResponseHandler
as soon
as the target system
(OMS/ERP)
has received/processed the order.
ExportFormatProvider
-
The
ExportFormatProvider
is a pipeline step executor used dynamically for the transform step -
The configuration is used to define the export format
-
As default, the Custom Template is used, which is editable in the backend with a Twig editor
-
Several export formats can be registered beside the Custom Template
TransportAdapterProvider
-
The
TransportAdapterProvider
is a pipeline step executor that is used dynamically for the transport step -
It gets used to define the export functionality and workflow
-
By default, the file system gets used
-
Besides the file system, other transport paths can get registered, e.g., through API or DB
ResponseHandlerProvider
-
The
ResponseHandlerProvider
is a pipeline step executor used dynamically for the handle response step -
The configuration manages responses regarding receipt and processing of a target system (OMS/ERP)
-
By default, a JSON file gets expected on the target directory of the file system, which has a reference to the external id
-
Besides the default JSON format, other formats like XML can get registered
-
Managing the responses is optional
NotificationHandlerProvider
-
The
NotificationHandlerProvider
is a pipeline step executor used dynamically for the handle notification step -
The configuration gets used to notify about responses and information
-
In addition to the default email notification, other notifications such as loggers can get registered
-
The notifications are optional and disabled by default
Reservation Handling
With the Reservation Handling feature in the Pacemaker Order Export, it is easy to define by configuration when stock levels are adjusted regarding product stock reservations.
If Pacemaker Order Export has been configurated to revoke the reservations through Pacemaker, then a separate step is created in the pipeline, which performs this stock/reservation revocation during execution.
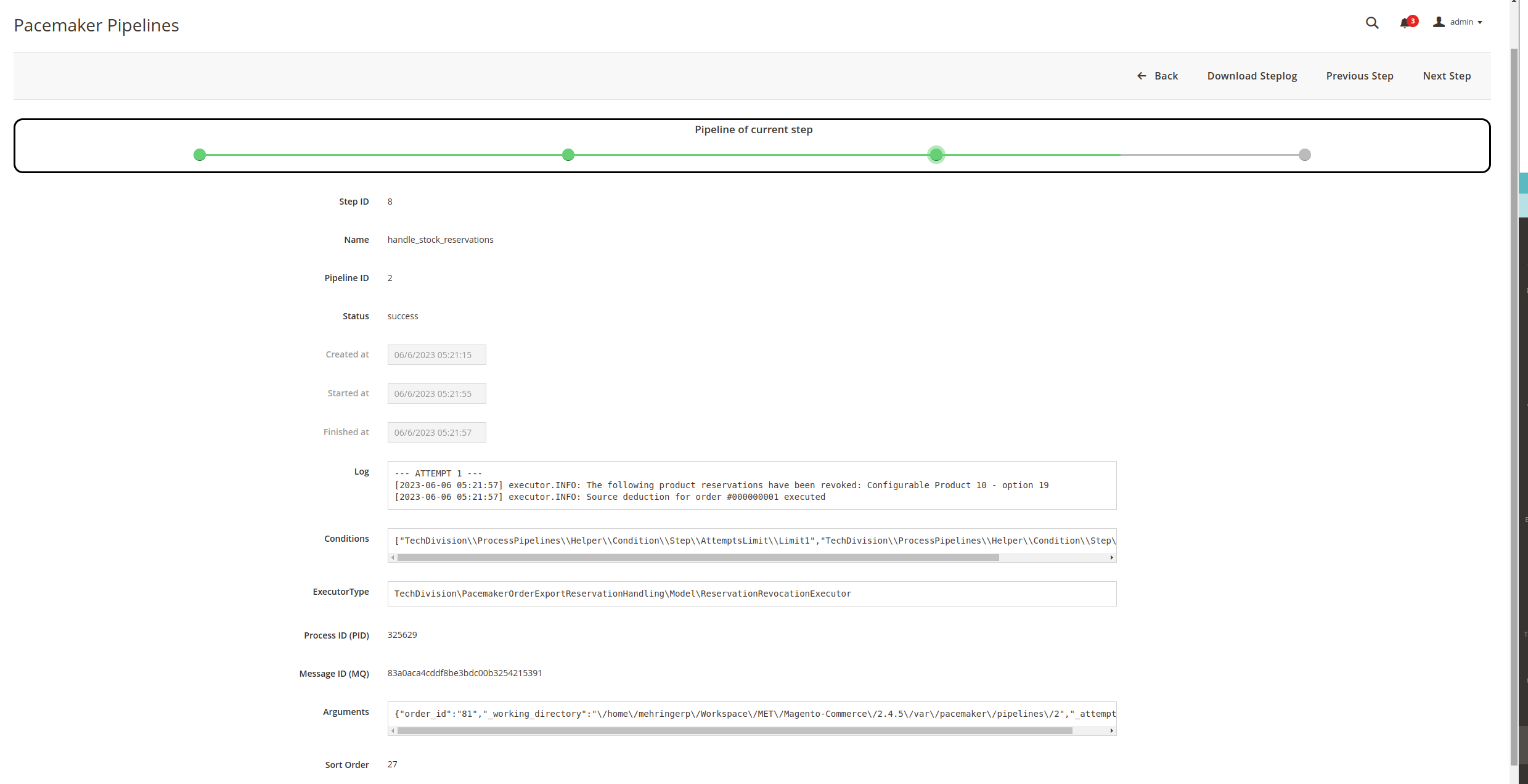
Technically, the stock is corrected and the reservation is resolved by an offsetting entry.

The following configuration settings are available:
Use Magento Standard
This is the default setting. Magento takes care of the stock/reservation revocation when creating shipments, creditmemos or cancellations.
Pacemaker does not generate a step in the order export pipeline and therefore does not execute any logic.
After Transport Step
This is where Pacemaker now intervenes in the reservation handling.
A step is created in the order-export pipeline after the transport step (export), which ensures that the reservation of the ordered products is resolved after the export.
The system only checks whether the transport step has been executed and not what status it has.

Depending on successful Transport Step
This option is similar to the previous one, but it also checks for a successful status of the transport step.
Depending on the export response
This option generates the reservation handling step after the response step instead of creating the step after the transport step. This is also checked for successful processing.
It should be noted here that a response handler must be configured. |

Order Status Payment Adjustment
With the Order Status Payment Adjustment feature in {label-pacemakerl} Order Export, it is easy to define by configuration when the order should get canceled based on the payment method/status and a user-defined time.
If Order Status Payment Adjustment gets configured to cancel orders that meet the conditions, a separate pipeline with a step gets created to cancel orders during execution.
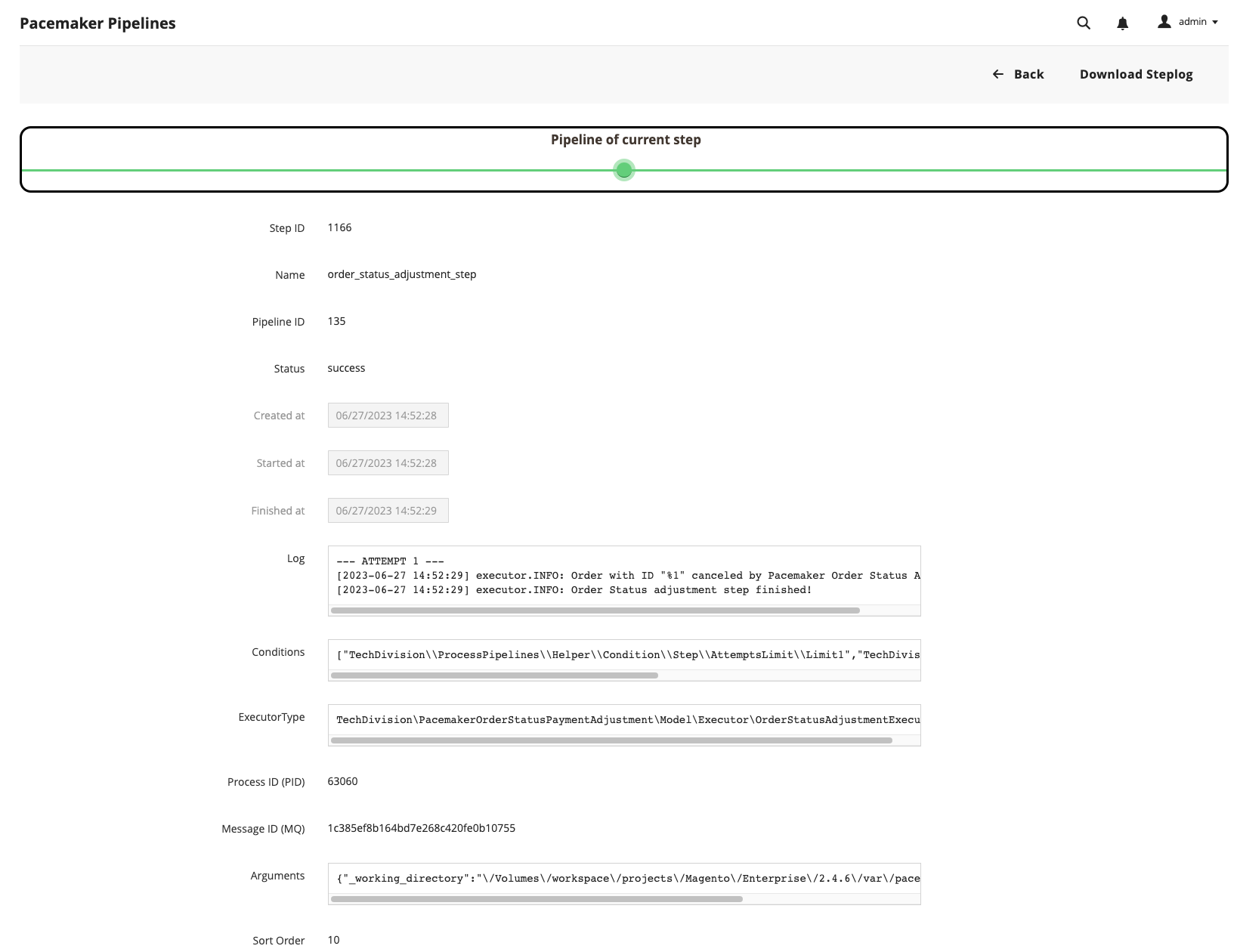
Technically, the status of the order gets set to canceled
.

The following four settings are available to configure the cancellation of orders. Only if all of the four settings apply to an order will it be canceled.
Payment Method
- This setting restricts orders cancellation to those with the selected payment method:
-
-
Any payment method is available in the shop can get chosen
-
Order Status to cancel
- This setting restricts orders cancellation to those with the selected order status:
-
-
It can get selected from the values
pending
andprocessing
-
Cancel order only if exported
This setting determines whether the cancellation of orders is limited to those exported by {label-pacemakerl} or if it also includes orders that have yet to get exported.
When the option gets set to Yes
, only orders listed in the pacemaker_order_export table with a value of 1
in the is_exported column will be considered.
Time Period
This setting limits the cancellation of orders to those whose creation date, when added within the specified period, is less than the current date and time.
The period can get specified in minutes, hours, days, weeks, months, and years.
Scheduling the pipeline by cron expression
The pipeline creation can get scheduled using a cron expression.
By default, the pipeline gets created at most once per minute.
If the heartbeat is executed twice per minute, with the default setting, the pipeline will get built only during the first execution and not during the second.
Any valid cron expression can get specified.